Documentation
Everything you need to know about integrating and customizing the Tadata MCP
Getting Started
Get your API equipped with the Model Context Protocol in minutes. Our JavaScript SDK makes it simple to connect with just a few lines of code.
Note: Works with Express, NestJS, Fastify, and other Node.js frameworks. For the full list of supported frameworks, see the documentation below.
Installation
Install the Tadata JavaScript SDK using your preferred package manager:
npm install @tadata-js/sdk
# or
yarn add @tadata-js/sdk
# or
pnpm add @tadata-js/sdk
Install the Tadata JavaScript SDK.
Quickstart
Deploy a Model Context Protocol (MCP) server with your OpenAPI specification:
import { Tadata, OpenApiSource } from '@tadata-js/sdk';
// Initialize with your API key
const tadata = new Tadata({
apiKey: process.env.TADATA_API_KEY,
});
// Create an OpenAPI source from JSON file
const source = await OpenApiSource.fromFile('./acme-openapi.json');
// Deploy the MCP server
const deployment = await tadata.mcp.deploy({
spec: source,
apiBaseUrl: 'https://acme.com/api',
});
console.log(`Deployed MCP server: ${deployment.url}`);
Basic example showing how to deploy an MCP server.
Advanced Configuration
Configure the SDK with advanced options for production use:
import { Tadata, OpenApiSource, ApiVersion } from '@tadata-js/sdk';
// Initialize the SDK with advanced options
const tadata = new Tadata({
apiKey: process.env.TADATA_KEY!,
version: ApiVersion.V_05_2025, // Optional: specify API version
logger: pino(), // Optional: use custom logger
});
// Create an OpenAPI source from JSON file
const source = await OpenApiSource.fromFile('./acme-openapi.json');
// Deploy the MCP server with advanced configuration
const deployment = await tadata.mcp.deploy({
spec: source,
apiBaseUrl: 'https://acme.com/api',
name: 'Acme API', // Optional
// Optional: Configure authentication handling
authConfig: {
passHeaders: ['authorization'], // Headers to pass through
passQueryParams: ['api_key'], // Query params to pass through
},
});
console.log(`Deployed Model Context Protocol (MCP) server: ${deployment.url}`);
Advanced SDK configuration with custom logging and authentication handling.
OpenAPI Source Formats
The SDK supports multiple ways to provide your OpenAPI specification:
// From a JSON file
const source = await OpenApiSource.fromFile('./openapi.json');
// From a JSON string
const source = OpenApiSource.fromJson(jsonString);
// From a JavaScript object
const source = OpenApiSource.fromObject({
openapi: '3.0.0',
info: { title: 'Example API', version: '1.0.0' },
paths: { /* ... */ }
});
Different methods to create an OpenAPI source for your MCP server.
Error Handling
The SDK provides specific error classes for better error handling:
import { SpecInvalidError, AuthError, ApiError, NetworkError } from '@tadata-js/sdk';
try {
await tadata.mcp.deploy({ /* ... */ });
} catch (error) {
if (error instanceof SpecInvalidError) {
console.error('Invalid OpenAPI spec:', error.message, error.details);
} else if (error instanceof AuthError) {
console.error('Authentication failed:', error.message);
} else if (error instanceof ApiError) {
console.error('API error:', error.message, error.statusCode);
} else if (error instanceof NetworkError) {
console.error('Network error:', error.message);
} else {
console.error('Unexpected error:', error);
}
}
Using specific error classes to handle various error scenarios.
Supported JavaScript Frameworks
Tadata's MCP SDK currently supports the following javascript frameworks:
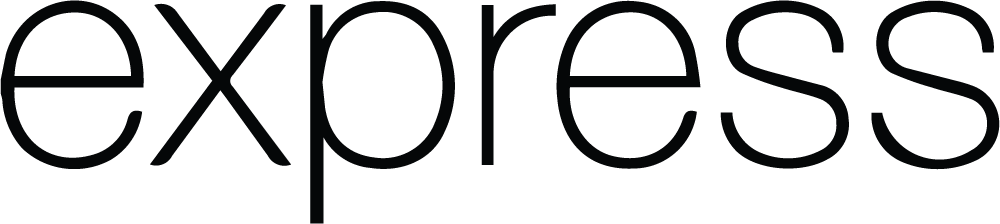
Express.js
Our primary Node.js framework with complete JavaScript SDK integration.
NestJS
Complete support for NestJS applications through our JavaScript SDK.
Fastify
Complete support for Fastify applications.
More Frameworks
We're actively expanding our javascript framework support.
Don't see your framework listed? Contact us to request support for additional frameworks.
How It Works
The Problem We're Solving
Connecting LLMs like ChatGPT, Claude, and Gemini to your application's API has traditionally required:
- ✗Manual creation of function specifications for every API endpoint
- ✗Maintaining separate OpenAI, Anthropic, and Google AI integrations
- ✗Building complex middleware for authentication and validation
- ✗Continuous updates when your API changes
Our Solution
Automatic MCP Generation
We automatically convert your API specifications into MCP-compatible endpoints, making them instantly accessible to AI clients.
Universal Compatibility
One integration works with all major AI models and tools like Cursor, ChatGPT plugins, and more.
Hosted Infrastructure
We handle the hosting, scaling, and maintenance of your MCP server so you don't have to.
Automatic Syncing
As your API evolves, your MCP automatically updates to reflect the changes.
How It Works in 3 Steps
- 1
Install our SDK
Add our lightweight JavaScript SDK to your existing API project.
- 2
Deploy your API
Use our SDK to deploy your OpenAPI specification as an MCP server.
- 3
Done!
Your API is now accessible via the Model Context Protocol, ready to be called by AI tools and assistants.
Ready to get started?
Deploy your MCP in seconds with no complex infrastructure.